SVG Logo Stroke Animation
Demo
How to
- Prepare Logo and Logo Stroke svg.
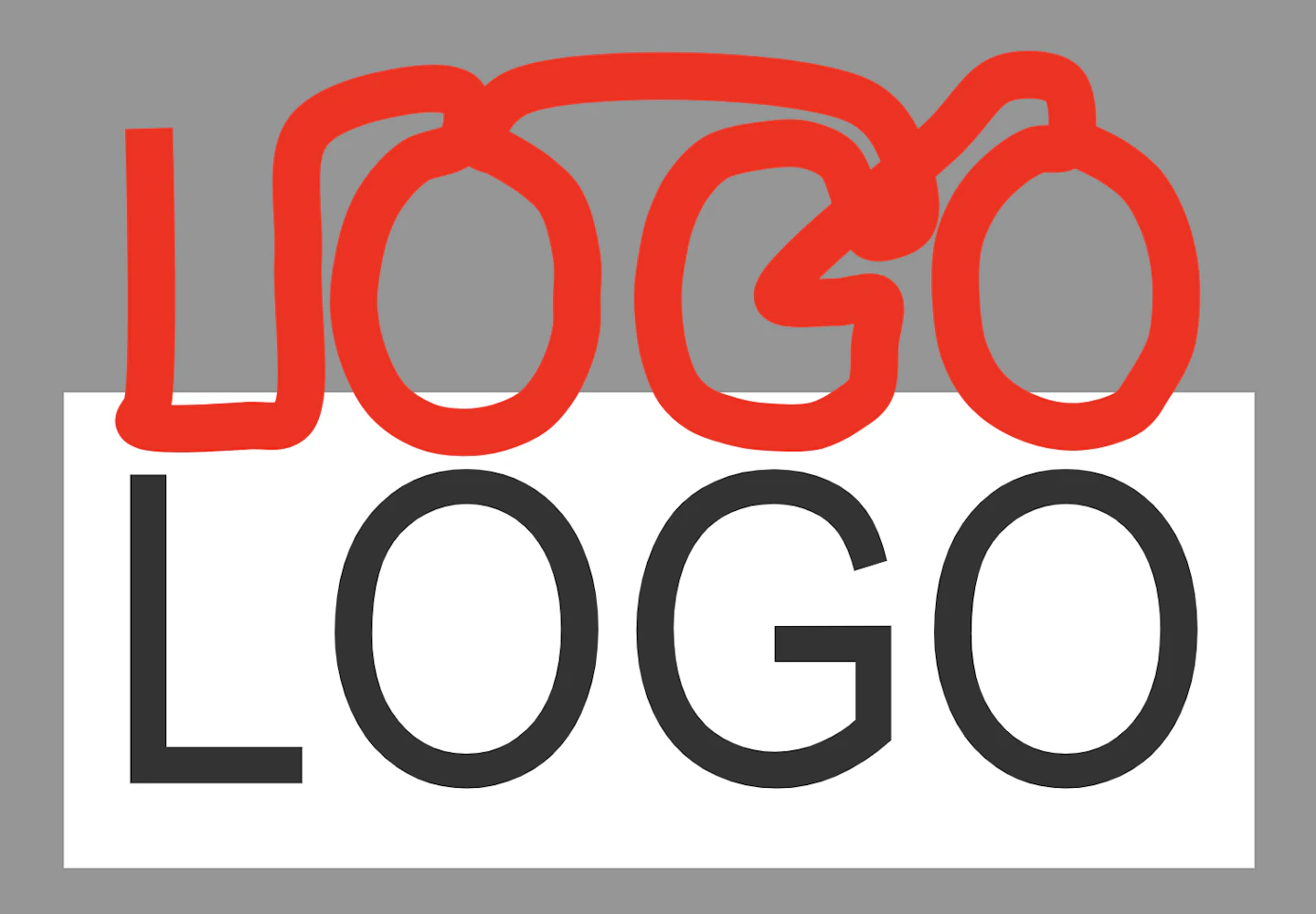
- Put logo mask under
<defs>
tag - Put logo stroke and set mark.
- Get stroke length by using
[path_element].getTotalLength()
- Set stroke dash array as the total length.
- Set animation by using the total length.
vue
<script setup lang="ts">
const strokeEl = ref<HTMLElement>();
onMounted(() => {
console.log('Stroke length: ', strokeEl.value.getTotalLength());
});
const isAnimate = ref(false);
const onClick = () => {
isAnimate.value = true;
};
const onAnimationEnd = () => {
isAnimate.value = false;
};
</script>
<template>
<svg viewBox="500 0 500 200" xmlns="http://www.w3.org/2000/svg">
<defs>
<mask id="logo-mask">
<!-- logo SVG -->
<g>
<path d="..." />
</g>
</mask>
</defs>
<g mask="url(#logo-mask)">
<!-- One lined stroke -->
<path
ref="strokeEl"
class="stroke"
d="..."
/>
</g>
</svg>
</template>
<style scoped>
@keyframes logo-ani {
from {
stroke-dashoffset: 1876;
}
to {
stroke-dashoffset: 0;
}
}
.stroke {
stroke-width: 20px;
stroke: white;
fill: none;
stroke-dasharray: 1876;
animation: logo-ani 2s linear;
</style>